- If the attribute required is included in an HTML input element
with type="text", a form that includes this element cannot be submitted
unless the input element is not empty. Write a form and an Express
project to test this. Answer:
--------------------------------------
// Source code file: main.js
const express = require("express");
const app = express( );
app.set("view engine", "ejs");
app.get("/input_form", (req, res) => {
res.render("input_form");
});
app.get("/process_form", (req, res) => {
var fname = req.query.fname;
res.render("process_form", {fname: fname});
});
app.listen(3000, ( ) => {
console.log("Listening on Port 3000.");
});
--------------------------------------
<!DOCTYPE html>
<!-- Source code file: views/input_form.ejs
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Input Form Example</title>
</head>
<body>
<h1>Input Form Example</h1>
<form name="form" action="/process_form" method="get">
<label>Enter the First Name:</label><br>
<input type="text" name="fname" required><br><br>
<input type="submit" value="Submit First Name">
</form>
</body>
</html>
--------------------------------------
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Process Form</title>
</head>
<body>
<h1>Process Form</h1>
<p>First Name: <%= fname %></p>
</body>
</html>
--------------------------------------
- Write an Express app that inputs student information on a form like
this:
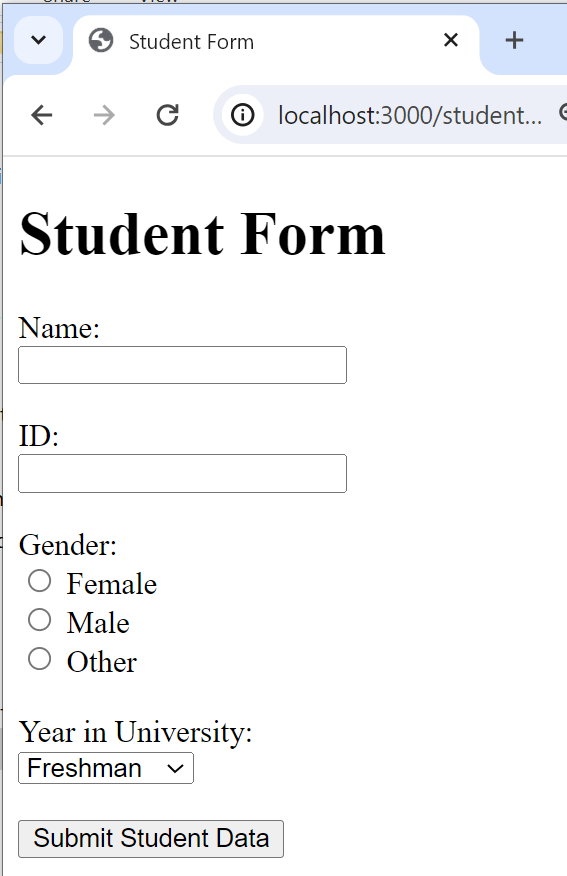
When this form is submitted, a view displaying the information received
by the server is shown, for example:
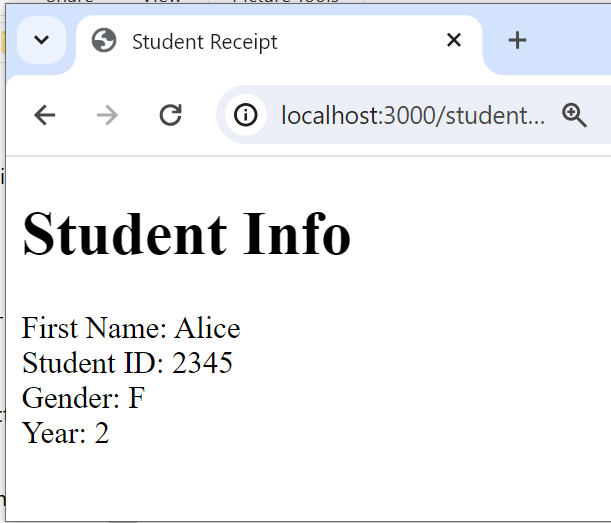
Answer:
--------------------------------------
// Source code file: main.js
const express = require("express");
const app = express( );
app.set("view engine", "ejs");
app.get("/student_form", (req, res) => {
res.render("student_form");
});
app.get("/student_receipt", (req, res) => {
var student_data = req.query;
res.render("student_receipt",
{ student_data: student_data });
console.log(student_data);
});
app.listen(3000, ( ) => {
console.log("Listening on Port 3000");
});
--------------------------------------
<!DOCTYPE html>
<!-- Source code file: views/student_info.ejs -->
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Student Form</title>
</head>
<body>
<h1>Student Form</h1>
<form name="form1" action="/student_receipt" method="get">
<label>Name:</label><br>
<input type="text" required name="firstName"><br><br>
<label>ID:</label><br>
<input type="number" required name="studentId"><br><br>
<label>Gender:</label><br>
<input type="radio" name="gender" value="F"> Female<br>
<input type="radio" name="gender" value="M"> Male<br>
<input type="radio" name="gender" value="X"> Other<br><br>
<label>Year in University:</label><br>
<select name="year">
<option value="1">Freshman</option>
<option value="2">Sophomore</option>
<option value="3">Junior</option>
<option value="4">Senior</option>
</select><br><br>
<input type="submit" value="Submit Student Data">
</form>
</body>
</html>
--------------------------------------
<!DOCTYPE html>
<!-- Source code file: student_receipt.ejs -->
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Student Receipt</title>
</head>
<body>
<h1>Student Info</h1>
<p>First Name: <%= student_data.firstName %> <br>
Student ID: <%= student_data.studentId %> <br>
Gender: <%= student_data.gender %> <br>
Year: <%= student_data.year %></p>
</body>
</html>
--------------------------------------
- InputElements Example: Test these other
values of the HTML input element type="text":
text number password date range color
Use an alert box to display the
the value of each input element after user input and button click. Here is the
source code for testing an input element with type="text":
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Test Input Element</title>
<script>
function showValue( ) {
var input1 = document.getElementById("in1");
var value1 = input1.value;
alert(`Value of input element: ${value1}`);
}
</script>
</head>
<body>
<h2>Test Input Element</h2>
<input type="text" id="in1"><br><br>
<button onclick="showValue( );">
Show Value of Input Element
</button>
</body>
</html>
- Start with this array of object literals:
var pets =
{
ID1001: { name: 'Sadie', animalType: 'dog', age: 3 },
ID1009: { name: 'Coco', animalType: 'cat', age: 4 },
ID1013: { name: 'Slinkie', animalType: 'snake', age: 2 },
ID1016: { name: 'Thumper', animalType: 'rabbit', age: 2 },
ID1027: { name: 'Max', animalType: 'dog', age: 5 },
ID1029: { name: 'Duke', animalType: 'dog', age: 7 },
};
- How can you determine if an object contains a specified key, for example
ID1016?
Answer: use the in operator:
console.log('ID1382' in persons);
// Output;
false
console.log('ID999' in persons);
// Output:
true
- Write a for loop that computes the average age of the dogs. Answer:
var sum = 0.0;
var count = 0;
for(let id in pets) {
if (pets[id].animalType == "dog") {
sum += pets[id].age;
count++;
}
}
console.log(sum / count);
- Look at the TestEjs5 Example. Modify it so that the form
uses method="post". Here is are the modified source code files. Test them.
Here are the source code files:
-------------------------------------------------
// Display a name obtained from an input form with
// method=GET
// Source code file: main.js
const express = require("express");
const app = express( );
app.use(express.urlencoded({extended: false}));
app.set("view engine", "ejs");
app.get("/contact_info", (q, r) => {
r.render("contact-info");
});
app.post("/contact_receipt", (q, r) => {
console.log(q.body);
r.render("contact-receipt", q.body);
});
app.listen(3000, ( ) => {
console.log("Listening on port 3000.");
});
-------------------------------------------------
// Source code file:
<!DOCTYPE html>
<!-- Source code file: contact-info.ejs -->
<html lang="en">
<head>
<meta charset="UTF-8">
<title>TestEjs2 Example</title>
</head>
<body>
<h2>TestEjs5 Example</h2>
<form name="form1"
action="/contact_receipt"
method="post">
<label for="fname">First Name:</label><br>
<input type="text" name="fname"><br><br>
<label for="lname">Last Name:</label><br>
<input type="text" name="lname"><br><br>
<label for="phone">Phone:</label><br>
<input type="text" name="phone"><br><br>
<label for="email">Email:</label><br>
<input type="text" name="email"><br><br>
<input type="submit" value="Submit Contact Info">
</form>
</body>
</html>
-------------------------------------------------
// Source code file:
<!DOCTYPE html>
<!-- Source code file: contact-receipt.ejs -->
<html lang="en">
<head>
<meta charset="UTF-8">
<title>TestEjs5 Example</title>
</head>
<body>
<h1>TestEjs5 Example</h1>
<p>Your contact information has been received<br>
Thank you for your information.</p>
<table>
<tr>
<th>First Name</th> <th>Last Name</th>
<th>Phone</th> <th>Email</th>
</tr>
<tr>
<td><%= fname %></td> <td><%= lname %></td>
<td><%= phone %></td> <td><%= email %></td>
</tr>
</table>
</body>
</html>
------------------------------------------------